What is a switch?This subject seems so simple that is barely warrants dedicating a lesson to it right?
I'm sure you already know what a switch is, you probably used one to turn on the computer that you're using to read this on, and you use several switches to type the address into the address bar of your browser, you used one to click a link to get here, you use switches when you turn on your car, turn on the lights, work an electric kettle etc. You know what a switch is, you use them all the time.
But there are a few terms and a few considerations that I thought it'd be good to go over.
A simple switch is just an on/off device, it lets you make or break an electrical connection. consider it as two pieces of wire, you either hold them together or apart, the only difference in that in a switch there are some bits that hold the wires for you.
A simple thing that you can do to make a switch it use a block of wood, a paper clip and two thumb tacs (the brass kind, not plastic.)
You attach the wire to the spike of the drawing pin by wrapping the bare end around it, then slot this through the drawing pin and push it in the piece of wood, then you wrap some wire round the spike of your second drawing pin and out this about the length of the drawing pin away from the first pin.
Now you can slide the paper clips over the second pin to "make" the circuit, or slide it off the drawing pin to break the circuit.
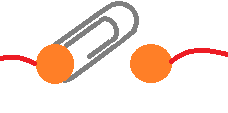
The symbol for a switch is not too dissimilar to what is seen above.
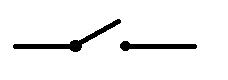
Kinda looks like that paper clip assembly huh?
PolesPoles refer to the amount of connections that can be made.
A simple on off switch that has a single input and a single output is a single pole switch.
Like a light switch, any switch with a single input is a single pole switch, regardless of the amount of ways the switch can be flipped or turned.
ThrowThrow refers to the amount of outputs that a switch has, so if a switch has output B or output B it's going to be double throw, if it's got three output contacts it'll be triple throw.
However, after triple we don't often us quadruple, quintuple, sextuple, we'd just say 4-way, 5-way and 6-way etc.
SymbolsThe circuit symbol for the single pole single throw (SPST) switch was pictured above, here it is again:
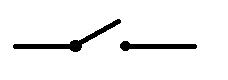
The double pole single throw (DPST) switch has two switches encased in the same packaging, and is actuated by the same level or button:
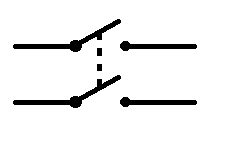
The single pole double throw (SPDT) switch has a single input and a choice of either output A or B:
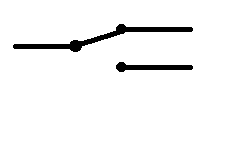
The double pole double throw switch has a circuit symbol similar to this, but obviously with two switches marked.
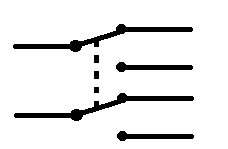
You can see that these switches are infinity stackable. you can have 6pole double throw switches just by stacking more and more switches.
MomentaryMomentary means that the switch returns to it's off position after you release your finger, it's got a spring in it that pushes the button back up again. like a horn in a car.
LatchingLatching means that when you push a switch down, it stays down, until you push it again, a bit like one of those pens where you click once to extend the pen to write, and click a second time to put the switching part of the pen away again.
Normally OpenNormally open switches are switches where the contacts are usually apart, you push to make the circuit, you push to connect wires inside the switch, again like a car horn or a doorbell.
Normally ClosedNormally closed switches are ones where the circuit is normally complete and you push the switch to interrupt or break the circuit, your pushing the switch disconnects the wires inside. switches like this are like the window switches in burglar alarm systems.
PushFairly simple, a push switch is one that you push on.
ToggleA toggle switch has a toggle or knob sticking up, you flick it one one position to another.
RockerRocker switches are switches that rock from side to side to be on or off, a typical light switch in the UK is a rocker switch
SlideSlide switches are ones that you slide from side to side to turn on or off (or select the pole that you want to be on).
RotaryRotary switches are ones that you turn to select an output.
KeyKey switches require a key to turn them, like your car ignition, these switches are used as a safety or security feature, the key ignition stops anyone being able to just start your car, that's security. A key switch can be used to turn of power to a workshop stopping anybody but the keyholder from turning the power on, this means that the workshop cannot be used without a supervisor being present, increasing safety.
RatingJust as wires have a current rating, so do the contacts and internal part of a switch. contact ratings for small projects (switching using 9v battery) usually don't matter. However if you want to switch mains voltages ensure that the part that you buy is rated for the current that you're planning on switching, and ensure that it's rated for the voltage too, if you put too higher voltage through a switch that's not meant for it, then all that happens it that the voltage jumps between the contacts as a spark, so your switch doesn't actually switch anything!
Bouncing (and de-bouncing)Perhaps the hardest thing with switches is explaining the idea of bouncing.
basically, if you only intend on building analogue circuits you can switch off now ('scuse the pun.)
however those with digital desires should read on.
Switch bounce is a phenomenon where as you either open of close a switch as the contacts touch or slide into place the switch rapidly bounces between an on an off state before finally either switching on or off as you'd wanted. This is usually caused by two things, either the contacts inside the switch are made of springy metal, and the genuinely bounce when they are snapped into place. with other switches this can be caused by dirty contacts as they slide over each other, so you have clean metal touching making a circuit, then a spot of corrosion breaks the circuit before clean metal slides in again making a circuit.
This happens so fast that, while you can measure it on an oscilloscope you couldn't measure it with a volt meter, you couldn't perceive it in a light that you were switching.
but in digital circuits you're usually dealing with very fast components,
say for example you're making a counter system where you want to count people into a club, every time someone walks through the door you press a button. and a different button when they come out.
Lets say that you've counted in 100 people, the trouble is each time you pressed the switch it bounced. it changed rapidly, on, off, on, off, on, off, on. then you let go of the switch and it bounces again, off, on, off, on, off...
now instead of counting in 1 person (your circuit expects 1 switch press for 1 person) it's received what it sees as 6 switch presses. so even though there is only 100 people in your club, your counter says 600 and you're reached your fire limit for the building, you can't let people in because your counter says that there are already too many people and you loose money.
What if there is a fire, as you evacuate your switches don't bounce as much when you could people out, your counter said 100 in, but only says 95 out. now people are risking their lives looking for 5 people in a burning building.
Or worse, you manage to count 100 in successfully, but your switches are bouncing as you're counting people out, 99 people have left and your counter bounced and says 100 have. now some guy is burning to death, but nobody knows.
So, clearly for some projects de-bouncing switches is just good because it saves against false data, other times it's vital, and can put lives in danger if it's not done!
Thankfully it's quite simple to stop switching bounce, all you need to do is sample the switch input at a reasonably reduced rate.
So our on, off, on, off, on off, on bounces happen within say 100th of a second. all we need to do is reduce out sample rate, so say every 200ths of a second, or less, that way this bouncing or chattering happens outside of our sample time and we don't see it.